Launch your digital solution quickly by
building on a proven platform
Avoid reinventing the wheel; build on our enterprise-grade Content Services Platform or CSP. We have coded all the parts that are hard to code and a hassle to test so that you can save time and effort.
One system to integrate all - with Microservices
A Content Services Platform or CSP is ideal for integrating systems, especially ones otherwise hard to integrate. Build digital products and services based on our CSP and expose your content as Microservices using the Sense/Net API. Build customer, employee, and partner-facing solutions on any platform for any device.
Model everything as content
In our view, almost anything can be modeled as content. Building on a Content Services Platform with ready-made services saves much time in learning, design, implementation, and testing - leading to fast software delivery with very few bugs. The operation cost of a standard platform is also lower.
- QUIZ
- SUPPORT TICKET
- DISCUSSION
- Q&A
- ARCHIVE
- RECORD
- SAVED QUERY
- CALENDAR
- EVENT
- WHITEBOARD
- COMMUNITY
- ORDER
- SHOPPER
- BULLETIN
- WEBSITE
- MAP
- TECHNICAL DRAWING
- PIECE OF ART
- LISTS
- FORMS
- FORM FIELDS
- FILES AND FOLDERS
- SOCIAL MEDIA POST
- WORKFLOW
- BUSINESS PROCESS
- ANNOUNCEMENT
- WIKI ARTICLE
- PRESENTATION
- WORKFLOW
- MEASUREMENT
- MEDICAL RECORD
- BACKLOG
- MULTIMEDIA
- HANDHELD DEVICE
- MEMO
- NEWS ARTICLE
- REPORT
- ORG CHART
- COMPANY
- TEAM
- DEPARTMENT
- TOOL
- TELEPHONE
- USERS
- SECURITY FOOTAGE
- BOOK
- BUILDING
- PRINTER
- BLUEPRINTS
- LOGISTICS
- DATA
- CHAT
- MESSAGE
- OKR
- PROJECT
- USER GROUP
- WORKGROUP
- SERVER
- SHOPPING CART
- CUSTOMER
- USER
- SPREADSHEET
- USER PROFILE
- TASK
- DOCUMENT
- CONTRACT
- IMAGE
- VIDEO
- CA
- INVOICE
Focus on your business problem instead of coding basic plumbing
The Content Services Platform provides many services applicable to all content stored in the system. Once you learn to work with content using our microservice API and model any business entity as content, all of the services listed below are available to you, saving time and effort.
-
Taxonomy
Store your content in a tree structure to form a hierarchy and make administration and controlling access easy. Work with content in a similar way to working with folders and files.
-
Automatic GUI
Use the automatically generated administration interface for managing your custom content.
-
Microservices API
Any content type you define is automatically exposed as a set of Microservices. Access your content through the REST API from any application.
-
Odata Standard
Adhering to the OData standard helps you focus on your business logic while consuming RESTful APIs without having to worry about the various approaches to REST.
-
Content Type Definition
Define your content types using a simple description file called the Content Type Definition or CTD.
-
Unlimited Fields
Add any number of typed fields to a content type and have them all automatically indexed for querying and free text search.
-
Folders
Each content can be a container, similar to a folder in a file system, but unlike file systems, containers can also have metadata and attachments.
-
Attachment Fields
Attach documents or any binary files to content as fields. This allows you to model even complex content types. Popular document types such as Microsoft Office or PDF are built in.
-
Free Text Search
All fields are automatically indexed and are searchable with free text search, including documents or files attached.
-
Query
Query content with an easy-to-learn query language or standard REST queries adhering to the OData standard.
-
Saved Queries
Queries can be saved and run at any time by any user with the necessary permissions. Saved queries are stored as Content, as you probably have guessed by now.
-
Smart Folders
Create folders that do not contain actual content but represent the result of a query. Then, save time by relying on the automatic GUI to display folder content.
-
Content Type Inheritance
Use inheritance similar to OOP when defining Content Types. Save time by modeling content using an existing content type, even the built-in ones.
-
Built-in Content Types
Save time using the 25 popular content types that come out of the box with Sense/Net. You can also change them or derive your own ones using inheritance.
-
Document Preview
Preview popular documents in a web browser without any plugins or client software. Create plugins for your custom content types.
-
Office 365
Collaborate with others on Microsoft Office documents in Office 365, directly opening documents from Sense/Net.
-
Permissions
Rely on the entity-level permissions to protect and control access to content. Control who can see, edit, modify, or set permissions or approve versions with eight built-in permissions.
-
Permission Inheritance
Permission settings are inherited similarly to a file system. Set permissions to branches of the content tree or individually or in a mixed mode.
-
Deny Permissions
Handle exceptions to permission settings using the Deny permission, which overrides all other permission settings to the given user or group.
-
Custom Permissions
Create any business logic using any one of the 16 custom permissions. Custom permissions work super efficiently, just like built-in permissions.
-
User Management
Users are modeled as content, so manipulating them is the same as working with any other content. You can search, query, and even set permissions for users.
-
Security Groups
Set permissions to individuals or groups of users. Security groups are modeled as content, so they can be easily populated by users and manipulated the usual way.
-
Provisioning
By giving permissions to manipulate users or user groups and setting permissions, you can provision user management and access control management to business users.
-
Version Control
You can retain old versions of a content when a user updates them. Use approval and permissions for version control. Revert to an old version when needed.
Integrate third-party systems or build stand-alone applications
Integrate other systems by transferring data between the given system and the content repository. New applications can be built directly on the Sense/Net platform. In both cases, content is exposed through the Sense/Net API as Microservices, and an automatically generated GUI helps speed up development.
Integrate with any system, even outdated legacy systems
Integrate content from any external system in three steps: modeling the content, transferring it to the Content Services Platform, and building applications using its services.
Model your content by defining custom Content Types
Building an application on the Sense/Net Content Services Platform starts by modeling content. Then, by editing a simple Content Type Definition file, you can create your own Content Types; everything else is done automatically. All fields are indexed, permissions are applied, version control is in place, and everything is exposed as an OData-compliant Microservices API.
<?xml version="1.0" encoding="UTF-8"?>
<ContentType name="Car" parentType="GenericContent" handler="SenseNet.ContentRepository.GenericContent"
xmlns="http://schemas.sensenet.com/SenseNet/ContentRepository/ContentTypeDefinition" >
<DisplayName>Car</DisplayName>
<Description>Content type for storing information about cars</DisplayName>
<Icon>Car</Icon>
<Fields>
<Field name="CarType" type="ShortText">
<DisplayName>CarType</DisplayName>
<Description>Type of the car</Description>
</Field>
<Field name="Color" type="ShortText">
<DisplayName>Color</DisplayName>
<Description>The color of the car</Description>
</Field>
<Field name="Mileage" type="Number">
<DisplayName>Mileage</DisplayName>
<Description>The mileage of the car in MPG</Description>
</Field>
<Field name="TakenBy" type="Reference">
<DisplayName>Taken By</DisplayName>
<Description>The user who currently has the car</Description>
</Field>
<Field name="ManufactureDate" type="DateTime">
<DisplayName>Manufacture Date</DisplayName>
<Description>The car's manufacture date</Description>
<Configuration>
<DateTimeMode>Date</DateTimeMode>
</Configuration>
</Field>
</Fields>
</ContentType>
Work with your content through the REST API
After creating your own Content Type, you can start manipulating your content through the OData-compliant Microservices API. Create, read, update, delete, run queries, search, and set permissions with easy-to-learn standard commands.// Init state
const [ cars , setCars ] = useState([])
// This query retrieves all content items of type "Car" that have a property "Color" equal to "Red"
// and a property "Mileage" greater than 50 and a property "TakenBy" equal to null.
const carQuery= await repository.loadCollection({
path: '/Root/Content',
oDataOptions:{
select: [ "Id", "Name", "Type", "DisplayName", "Color", "Mileage" ]
query: "Type:'Car' AND Color:'Red' AND Mileage:>50 AND TakenBy:''"
},
})
// Update State
setCars( carQuery.results.d )
// Render Component
return (
<table>
<th>
<td>Car</td><td>Color</td><td>Mileage</td>
</th>
{cars .map((item) => (
<tr key={item.Id}>
<td>{item.DisplayName}</td>
<td>{item.Color}</td>
<td>{item.Mileage}</td>
</tr>
)}
</table>
)
// This query retrieves all content items of type "Car" that have a property "Color" equal to "Red"
// and a property "Mileage" greater than 50 and a property "TakenBy" equal to null.
const cars = await repository.loadCollection({
path: '/Root/Content',
oDataOptions:{
select: [ "Id", "Name", "Type", "DisplayName", "Color", "Mileage" ]
query: "Type:'Car' AND Color:'Red' AND Mileage:>50 AND TakenBy:''"
},
})
//Console Log the result
cars.forEach((item) => {
console.log("DisplayName:", item.DisplayName);
console.log("Color:", item.Color);
console.log("Mileage:", item.Mileage);
console.log("--------"); // Add a separator for clarity
});
// This query retrieves all content items of type "Car" that have a property "Color" equal to "Red"
// and a property "Mileage" greater than 50 and a property "TakenBy" equal to null.
const query = encodeURIComponent("$query=Type:'Car' AND Color:'Red' AND Mileage:>50 AND TakenBy:''");
const baseUrl = "https://yourserver/OData.svc/Root/Content";
const cars = await fetch(
`${baseUrl}?${query}`,
{credentials: "include"}
);
cars.forEach((item) => {
console.log("DisplayName:", item.DisplayName);
console.log("Color:", item.Color);
console.log("Mileage:", item.Mileage);
console.log("--------"); // Add a separator for clarity
});
// This query retrieves all content items of type "Car" that have a property "Color" equal to "Red"
// and a property "Mileage" greater than 50 and a property "TakenBy" equal to null.
store.dispatch(Actions.fetchContent(
path:"/Root/Content",
query:{"Type:'Car' AND Color:'Red' AND Mileage:>50 AND TakenBy:''"}
})
// This query retrieves all content items of type "Car" that have a property "Color" equal to "Red"
// and a property "Mileage" greater than 50 and a property "TakenBy" equal to null.
var request = new QueryContentRequest
{
ContentQuery = "Type:'Car' AND Color:'Red' AND Mileage:>50 AND TakenBy:''",
Select = new[] { "Id", "Name", "Type", "DisplayName", "Color", "Mileage" }
};
// Execute the query remotely and get the result as a collection of the desired type
var cars = await repository.QueryAsync<Car>(request, cancel);
// Display result as a simple table
Console.WriteLine($"Car\\tColor\\tMileage");
foreach (var car in cars)
{
Console.WriteLine($"{car.DisplayName}\\t{car.Color}\\t{car.Mileage}");
}
# This query retrieves all content items of type "Car" that have a property "Color" equal to "Red"
# and a property "Mileage" greater than 50 and a property "TakenBy" equal to null.
GET https://yourserver/OData.svc/Root/Content?$query=Type:'Car' AND Color:'Red' AND Mileage:>50 AND TakenBy:''
Save time by using the automatically generated GUI
As soon as you define a Content Type, an automatically generated GUI is at your service to manage that type of content. You can use the automatic GUI during development, administrative tasks, and as an end-user GUI to save time and money, especially in the MVP or prototype phase of software projects.
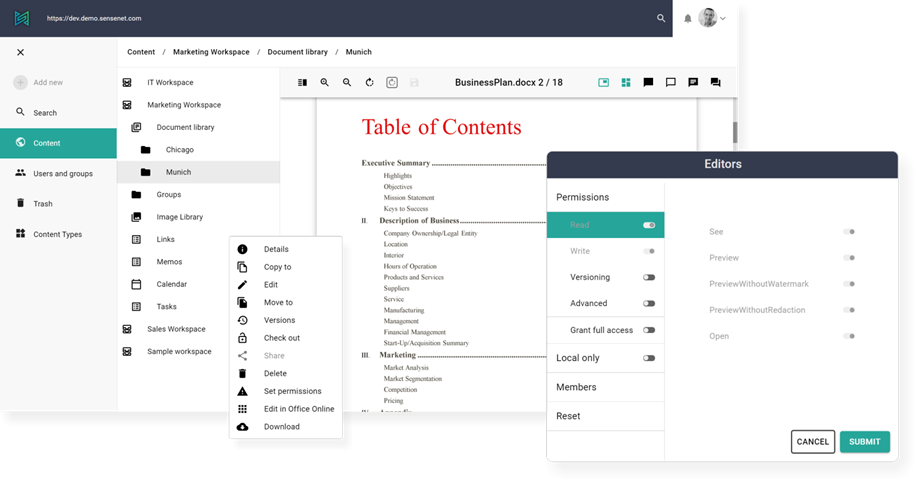
Economical to scale from SMB to Enterprise level
Sense/Net CSP is scalable from serving small-scale applications to serving millions of content to millions of users with relatively low hardware requirements. It also satisfies other enterprise-level non-functional requirements, such as performance, availability, fault tolerance, security, and many more.
-
100+ million content
We have live systems running with millions of content on standard servers under significant load and with complex permissions.
-
2 ms response time
Query and free text search response times remain low even under significant load querying millions of content.
-
Fintech level security
We have a track record of building and operating banking and fintech solutions with COBIT5 security standard.
-
99.999% SLA
The software supports all the technologies necessary for building systems with high availability.
Achieve high performance with our powerful search and query engine
Every content is automatically indexed upon creation or updating. An unlimited number of typed fields, including binary attachments, are available for query and search with a simple query syntax or on the built-in GUI. The performance of the Lucene-powered queries exceeds that of SQL servers.

Provide privacy and security with our powerful permission system
Set allow or deny permissions for individual users or groups on individual content, folders, or tree branches. Managing and visualizing the permission settings is available on the built-in GUI and with the REST API. Super-fast permission resolution is done in memory by a code we’ve been optimizing for years.
We support your development effort with custom services
Anything that can be outsourced saves time and allows you to focus on your business. From answering support tickets to completing projects, we are here to help your team. The Sense/Net CSP development team works closely with our Custom Software Solution development teams and Managed Cloud team to resolve issues quickly.
-
Tech Support
We provide tech support for development and operations at different SLA levels or even with dedicated staff.
-
Training
We provide installation, operation, architecture design, and development training with the Sense/Net CSP.
-
Consulting
Our consulting team is ready if you need advice or assistance in designing or implementing your system.
-
Infrastructure design
Our system engineers, who designed our cloud, design your infrastructure tailored to your requirements and budget.
-
System Architecture
Our senior architects and software engineers can help you model your data as content and optimize the software architecture.
-
Agile development
Our agile development teams are available for outsourcing short- and long-term, partial, or full-service development.
We have your back with regular updates and emergency bugfix services
We do our best to ship regular updates and bug fixes. We are also ready to support customers when disaster strikes, no matter what causes the disaster.
-
Regular Updates
We regularly ship updates and bug fixes to the Sense/Net platform at least every month.
-
Emergency Bugfixes
In case of a disaster, we cooperate to find a fix within a short time frame.
-
Disaster Assistance
We assist you in fixing or reinstalling your system when a disaster strikes.
Flexible licensing without legal complications
The Sense/Net CSP has three licensing models for enthusiasts, corporations, and product developers. In addition, we always give custom price quotes based on answering a few questions, usually followed by a short consultation to find the best order.
-
Open Source
The core services of the Sense/Net CSP are available as Open Source under the GPL license. However, we do not provide support services for the users of the Open-Source version.
-
Corporate License
The entire Sense/Net CSP can be licensed as a standalone product for an annual license fee or as part of the Cloud Services we provide for a monthly or yearly fee. Corporate licenses offer legal protection or indemnification. They also include some support services, while other services may be purchased for additional fees.
-
OEM Custom License
Sense/Net SCP can be licensed as part of a hardware or software product or service offering. We are open to custom licensing as well.
Focus on your business, outsource operation, maintenance, and support
Operate your CSP-based solution in our public cloud servers or a totally separated private cloud built specifically for your needs. For utmost privacy, we can install the system on your premises. In all cases, we provide consulting, design, and operation services.
-
Content Services Cloud
Rent one instance of the Sense/Net Content Services Platform and manage your content immediately without upfront investment or hassle in our public cloud infrastructure.
-
Private Cloud
Rent private virtual or physical servers or entire private cloud infrastructure for ultimate privacy and flexibility, hosted in one or both hosting facilities, totally separated from other clients.
-
Hybrid or On-premise Cloud
Custom-designed private cloud hosted in our facilities, or on your premises, or in both as a hybrid cloud solution. Partial or complete remote operation is an option.
-
Operation outsourcing
Our support and operation team of seasoned system engineers operate your system, from the operating system to the custom software level. It’s your choice how much responsibility you outsource.
Outsource CSP development and get results in a S.N.A.P.
We deliver Custom Software Solutions and digital products with a short time to market, high product-market fit, and superb ROI. To achieve this, we have combined our 25 years of CMS, ECM, and Content Services Platform experience with agile methodologies to form the Sense/Net Agile Process or S.N.A.P.
Trusted around the globe
Millions of content are stored and managed in Sense/Net CSP installations worldwide by companies of many sizes and industries.
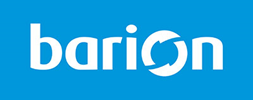
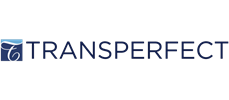
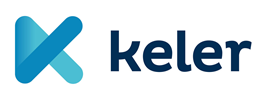

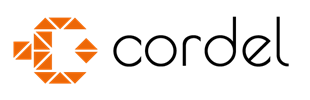
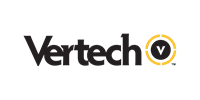
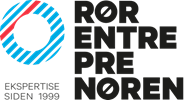
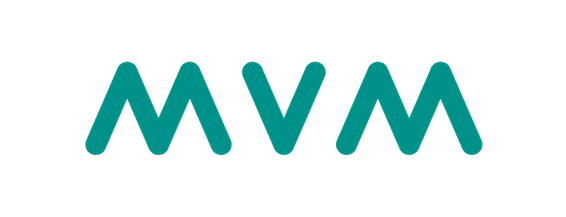
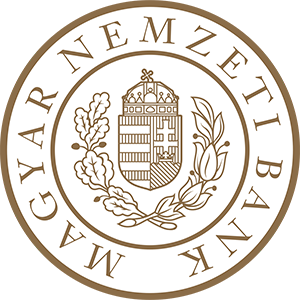
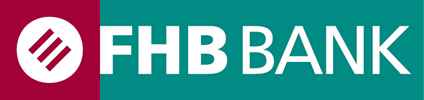
Brought to you by agile teams of seasoned content industry experts
This software is built by digital natives who strive for excellence and are passionate about supporting digitalization. Customer happiness is no cliché for us. We have experience from hundreds of content-related projects, some of which handle tens of millions of content, while others require banking-level security or have to withstand Black Friday level traffic every day.
Talk to our CSP experts
We are ready to help you evaluate Sense/Net CSP for your next software project or product. So book an appointment, and you can talk to IT experts instead of your typical salesperson.